Tesh-Clock
For my clock I wanted to create something that was not a direct analog to seconds, minutes, and hours. Instead I wanted to explore the idea of relative time, and describing time as it relates to how we experience it. This clock frames time as a finite amount per day that ticks down from 24 hours back to 0.
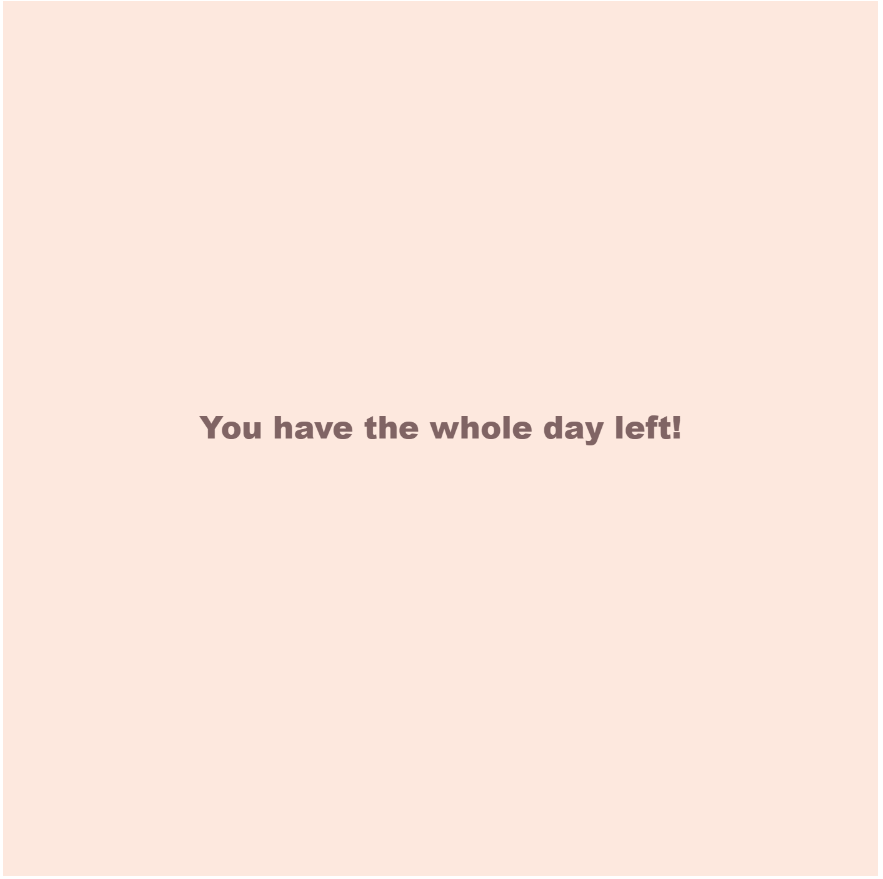
The clock then suggests different activities that you could feasibly do with the amount of time remaining in the day. I aggregated the lists myself by looking up the time it takes to do different activities and building them up in several arrays. The activities are also not centered around exact individual measures, but rather around more general times like sets of 5 minutes, half hours, 2 hours, etc.
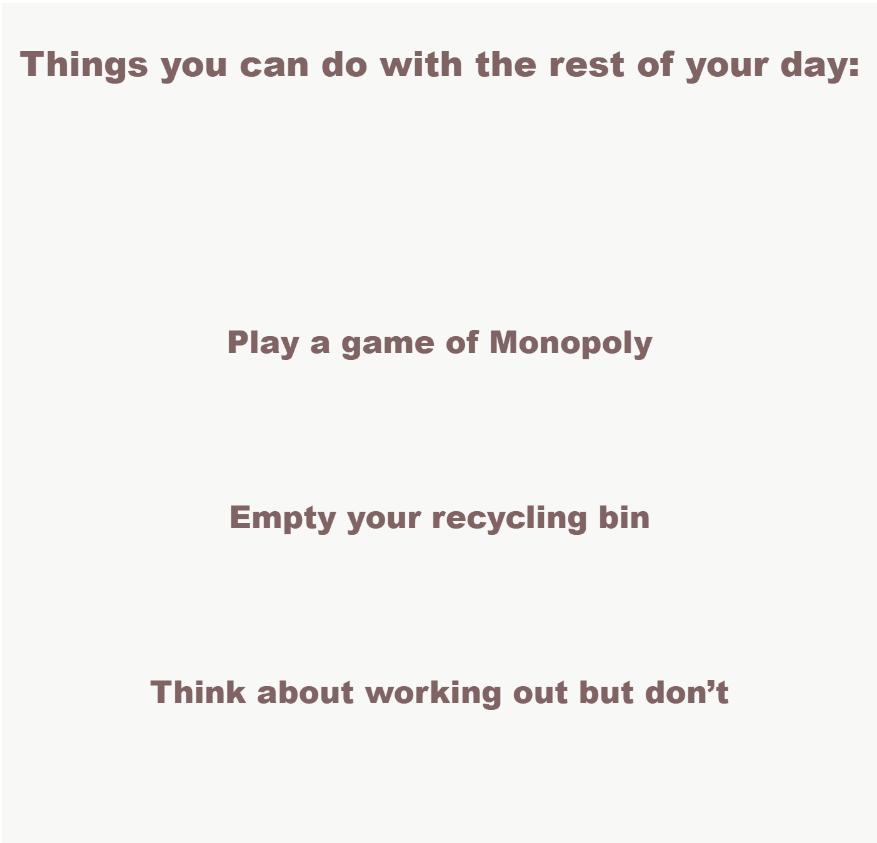
The clock uses these thresholds to decide when it would not be feasible to recommend things that take too much time, and once there is less than 2 out of 24 hours left in the day, goes into further granularity, referencing the individual minute scale more often. The clock additionally changes background color based on the real time, further hinting at them but never stating any numerical times.
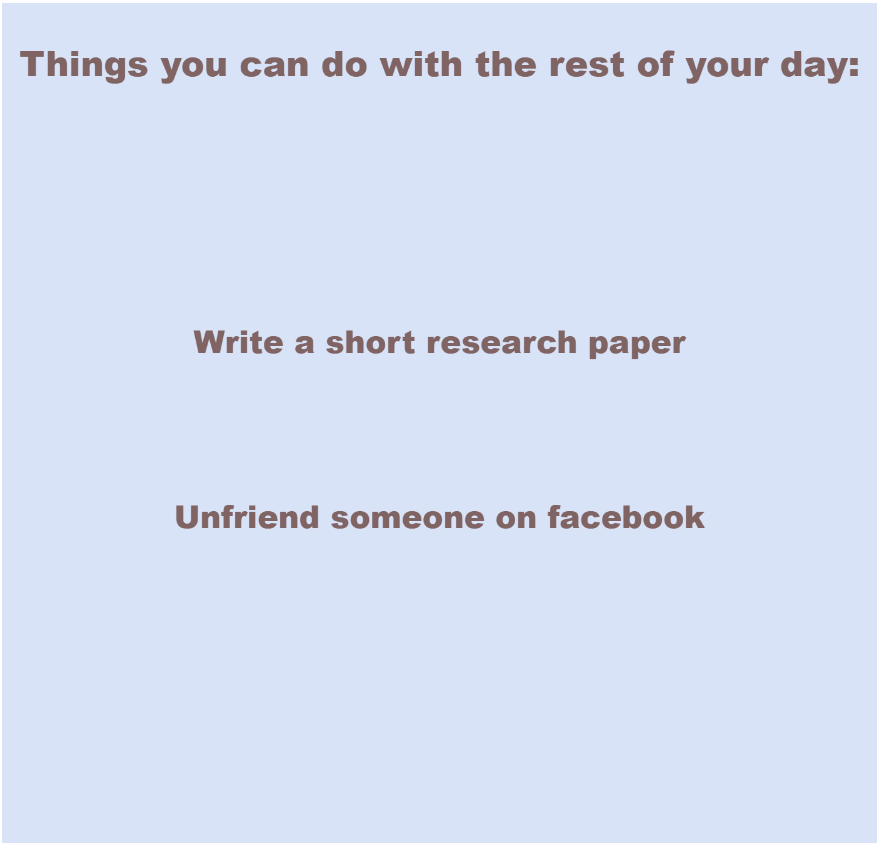
I would love to work more on this concept and create something more aesthetically pleasing that also convey’s the message I was going for. I also think there might be a bug or 2 that causes the program to freeze sometimes. Refreshing seems to help but I have not been able to diagnose what the problem is yet.
I was heavily inspired by the clock that Greg Vassallo made in 2015 while studying under Golan Levin. and I wanted to try out a different concept driving a similar idea.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 | var modMinSmall = 5; var modMinReg = 0; var modMinBig = 0; var modHr = 0; var modHrReg = 0; var minSmallTxtRange = ""; var minBigTxtRange = ""; var minRegTxtRange = ""; var hrTxtRange = ""; var hrRegTxtRange = ""; var min1 = []; var min5 = []; var min10 = []; var min30 = []; var hr1 = []; var hr2 = []; var hr3 = []; var hr4 = []; var hr5 = []; var hr6 = []; var hr8 = []; var hr12 = []; var hr14 = []; var hr16 = []; var hr18 = []; var hr24 = "You have the whole day left!"; var prevSec; var millisRolloverTime; var HPrev = -1; var MPrev = -1; //-------------------------- function setup() { createCanvas(700, 700); millisRolloverTime = 0; initializeTimePhrase(); textFont('Arial Black', [25]); textAlign(CENTER); modMinSmall = 0; modMinReg = 0; modMinBig = 0; modHr = 0; modHrReg = 0; } //-------------------------- function draw() { background(255, 255, 255); // textAlign(CENTER); // Fetch the current time var H = hour(); var M = minute(); var hColor = map(hour(), 0, 23, 0, 255); var mColor = map(minute(), 0, 60, 0, 255); var sColor = map(second(), 0, 60, 0, 255); background(hColor, mColor, sColor, 50); // //which minute text is displayed? //text("Min: " + M, width / 2, height / 2); if (H >= 2) { textFont('Arial Black', [28]); text("Things you can do with the rest of your day:", width / 2, height/12); textFont('Arial Black', [25]); //Larger Min Range if (60 - M > 30) { modMinBig = 30; if(MPrev != M &&M%modMinBig == 0){ minBigTxtRange = min30[int(random(min30.length))]; } } else if (60 - M > 10) { modMinBig = 10; if(MPrev != M &&M%modMinBig == 0){ minBigTxtRange = min10[int(random(min10.length))]; } } else if (60 - M > 5) { modMinBig = 5; if(MPrev != M && M%modMinBig == 0){ minBigTxtRange = min5[int(random(min5.length))]; } } //Smaller Min Range if (60 - M - modMinBig > 10) { modMinReg = 10; if(MPrev != M && M%modMinReg == 0){ minRegTxtRange = min10[int(random(min10.length))]; } } else if (60 - M - modMinBig > 5) { modMinReg = 5; if(MPrev != M && M%modMinReg == 0){ minRegTxtRange = min5[int(random(min5.length))]; } } else if (60 - M - modMinBig > 1) { modMinReg = 1; if(MPrev != M && M%modMinReg == 0){ minRegTxtRange = min1[int(random(min1.length))]; } } if (HPrev != H) { //Larger Hr Range if (24 - H > 18) { modHr = 18; hrTxtRange = hr18[int(random(hr18.length))]; } else if (24 - H > 16) { modHr = 16; hrTxtRange = hr16[int(random(hr16.length))]; } else if (24 - H > 14) { modHr = 14; hrTxtRange = hr14[int(random(hr14.length))]; } else if (24 - H > 12) { modHr = 12; hrTxtRange = hr12[int(random(hr12.length))]; } else if (24 - H > 8) { modHr = 8; hrTxtRange = hr8[int(random(hr8.length))]; } else if (24 - H > 6) { modHr = 6; hrTxtRange = hr6[int(random(hr6.length))]; } else if (24 - H > 5) { modHr = 5; hrTxtRange = hr5[int(random(hr5.length))]; } else if (24 - H > 4) { modHr = 4; hrTxtRange = hr4[int(random(hr4.length))]; } else if (24 - H > 3) { modHr = 3; hrTxtRange = hr3[int(random(hr3.length))]; } //Smaller Hr Range if (24 - H - modHr > 2) { modHrReg = 2; hrRegTxtRange = hr2[int(random(hr2.length))]; } else if (24 - H - modHr > 1) { modHrReg = 1; hrRegTxtRange = hr1[int(random(hr1.length))]; } } if ((60 - M) % modMinBig >= 0) { //text(min1[int(random(2))],140,22); text(minBigTxtRange, width / 2, height/5*4); } if ((60 - M) % modMinReg >= 0) { //text(min1[int(random(2))],140,42); text(minRegTxtRange, width / 2, height/5*3); } if ((H) % modHr >= 0) { //text(min1[int(random(2))],140,42); text(hrTxtRange, width / 2, height/5*2); } if ((H) % modHrReg >= 0) { //text(min1[int(random(2))],140,42); text(hrRegTxtRange, width / 2, height/5); } } else if (H >= 0) { // Midnight Case if (H == 0 && M == 0) { text(hr24, width / 2, height/2); } else { textFont('Arial Black', [28]); text("Things you can do with the rest of your day:", width / 2, height/12); textFont('Arial Black', [25]); if (MPrev != M) { //Larger Min Range if (60 - M > 30) { modMinBig = 30; minBigTxtRange = min30[int(random(min30.length))]; } else if (60 - M > 10) { modMinBig = 10; minBigTxtRange = min10[int(random(min10.length))]; } else if (60 - M > 5) { modMinBig = 5; minBigTxtRange = min5[int(random(min5.length))]; } //Smaller Min Range if (60 - M - modMinBig > 10) { modMinReg = 10; minRegTxtRange = min10[int(random(min10.length))]; } else if (60 - M - modMinBig > 5) { modMinReg = 5; minRegTxtRange = min5[int(random(min5.length))]; } else if (60 - M - modMinBig > 1) { modMinReg = 1; minRegTxtRange = min1[int(random(min1.length))]; } //Smallest Min Range if (60 - M - modMinBig - modMinReg > 5) { modMinSmall = 5; minSmallTxtRange = min5[int(random(min5.length))]; } else if (60 - M - modMinBig - modMinReg > 1) { modMinSmall = 1; minSmallTxtRange = min1[int(random(min1.length))]; } } if (HPrev != H) { //Smaller Hr Range if (3 - H - modHr > 2) { modHrReg = 2; hrRegTxtRange = hr2[int(random(hr2.length))]; } else if (3 - H - modHr > 1) { modHrReg = 1; hrRegTxtRange = hr1[int(random(hr1.length))]; } } if ((60 - M) % modMinBig >= 0) { text(minBigTxtRange, width / 2, height/5*4); } if ((60 - M) % modMinReg >= 0) { text(minRegTxtRange, width / 2, height/5*3); } if ((60 - M) % modMinSmall >= 0) { text(minSmallTxtRange, width / 2, height/5*2); } if ((H) % modHrReg >= 0) { text(hrRegTxtRange, width / 2, height/5); } } } textAlign(LEFT); fill(128, 100, 100); /*text("Hour: " + H, 10, 22); text("Minute: " + (60 - M), 10, 42); text("Second: " + S, 10, 62);*/ MPrev = M; HPrev = H; } function initializeTimePhrase() { //minute 1 min1[0] = "Floss your teeth"; min1[1] = "Back up your computer"; min1[2] = "Replace your toilet paper"; min1[3] = "Water your plants"; min1[4] = "Take your vitamins"; min1[5] = "Refill your soap dispenser"; min1[6] = "Send an email"; min1[7] = "Shake out a mat"; min1[8] = "Give someone a hug"; min1[9] = "Make your bed"; min1[10] = "Empty your recycling bin"; min1[11] = "Blink 20 times"; min1[12] = "Start a To-Do list"; min1[13] = "Get a glass of water"; min1[14] = "Hold your breath until you can’t anymore"; min1[15] = "Unfriend someone on facebook"; min1[16] = "Have an epiphany"; min1[17] = "Microwave hot chocolate"; min1[18] = "Wipe down your phone screen"; min1[20] = "Crack all your joints"; //minute 5 min5[0] = "Take a walk around the block"; min5[1] = "Return a quick phone call"; min5[2] = "Delete those apps you don’t use"; min5[3] = "Listen to a sick beat"; min5[4] = "Make one of those Tasty recipes"; min5[5] = "Chug a smoothie"; min5[6] = "Take an online quiz"; min5[7] = "Clean your computer desktop"; min5[8] = "Think about working out but don’t"; min5[9] = "Tell a really bad joke"; min5[10] = "Think of a good name for an \ninanimate object"; min5[11] = "Move clothes into the dryer"; //minute 10 min10[0] = "Learn to whistle"; min10[1] = "Learn 5 phrases in a new language"; min10[2] = "Make fancy oatmeal"; min10[3] = "Make a sub sandwich"; min10[4] = "Eat someone else's sandwich"; min10[5] = "Read a short article"; //minute 30 min30[0] = "Bake a cake from scratch"; min30[1] = "Take a selfie you actually like" min30[2] = "Update your LinkedIn profile \n#Networking"; min30[3] = "Work out at home"; min30[4] = "Hide your coworker's briefcase"; min30[5] = "Prank one of your friends"; min30[6] = "Have a quick shave"; //hours hr1[0] = "Take a nap" hr1[1] = "Watch a TV show" hr1[2] = "Swim a few laps" hr1[3] = "Take an indulgent bubble bath"; hr1[4] = "Go grocery shopping"; hr1[5] = "Get a haircut"; hr1[6] = "have a massage"; hr1[7] = "Clean out your refrigerator"; hr1[8] = "Rearrange your house"; hr2[0] = "Bleach and dye your hair"; hr2[1] = "Do a round of bowling"; hr2[2] = "Get your wisdom teeth removed"; hr2[3] = "Make fresh pasta from scratch"; hr2[4] = "Watch a summer blockbuster"; hr3[0] = "Play a game of Monopoly"; hr3[1] = "Watch a game of American Football"; hr3[2] = "Slowcook a meal in a crock pot"; hr4[0] = "Play a fun game of Risk"; hr4[1] = "Play Journey from start to finish"; hr4[2] = "Drive from Washington DC to a beach"; hr4[3] = "Go through almost all the exhibits \nat an art museum."; hr5[0] = "Run a decent marathon"; hr5[1] = "Make beef stew from start to finish"; hr6[0] = "Climb Mount Fuji from its base"; hr6[1] = "Go to public school, but skip the last class"; hr8[0] = "Play a really long and terrible game of Risk"; hr8[1] = "Pick 9 bins of apples"; hr12[0] = "Watch the entirety of the \nLord of The Rings trilogy"; hr12[1] = "Work an average day on the farm"; hr14[0] = "Finish an Ironman competition"; hr14[1] = "Get really good sleep for an 13 year old"; hr16[0] = "Take a bus from NYC to Atlanta, Georgia"; hr16[1] = "Write, set up, and perform a play"; hr16[2] = "Drive from Denver Colorado to Phoenix Arizona"; hr16[3] = "Do a week's worth of work as a part-time intern"; hr18[0] = "Fly from LA to Singapore"; hr18[1] = "Take a bus though Central America"; hr18[2] = "Write a short research paper"; } |