I like letters. Letters are fun. So are teeth (unless someone is eating you). So for this assignment, I chose to channel the letter O’s feral nature and gave it teeth.
[iframe src=”http://www.anitype.com/entry/agtzfmFuaXR5cGVjb3IUCxIHbGV0dGVycxiAgIDA2Z2QCQw/” width=”620″ height=”360″]
http://www.anitype.com/entry/agtzfmFuaXR5cGVjb3IUCxIHbGV0dGVycxiAgIDA2Z2QCQw/
When I first approached this assignment, I was fascinated by the one letter animation process. In my mind, animating letters was always done in the form of taking a word and giving it a property, such as, the word “dog” would behave like a dog, and the word “fly” would grow wings and move around a screen.
I ended up choosing the letter O and began to think of what kind of properties it would have. Automatically, I thought of it as a mouth, but I designed it in a way so that it would behave like a predator. At first, it looks harmless, but after a moment, it grows long teeth that form the letter O.
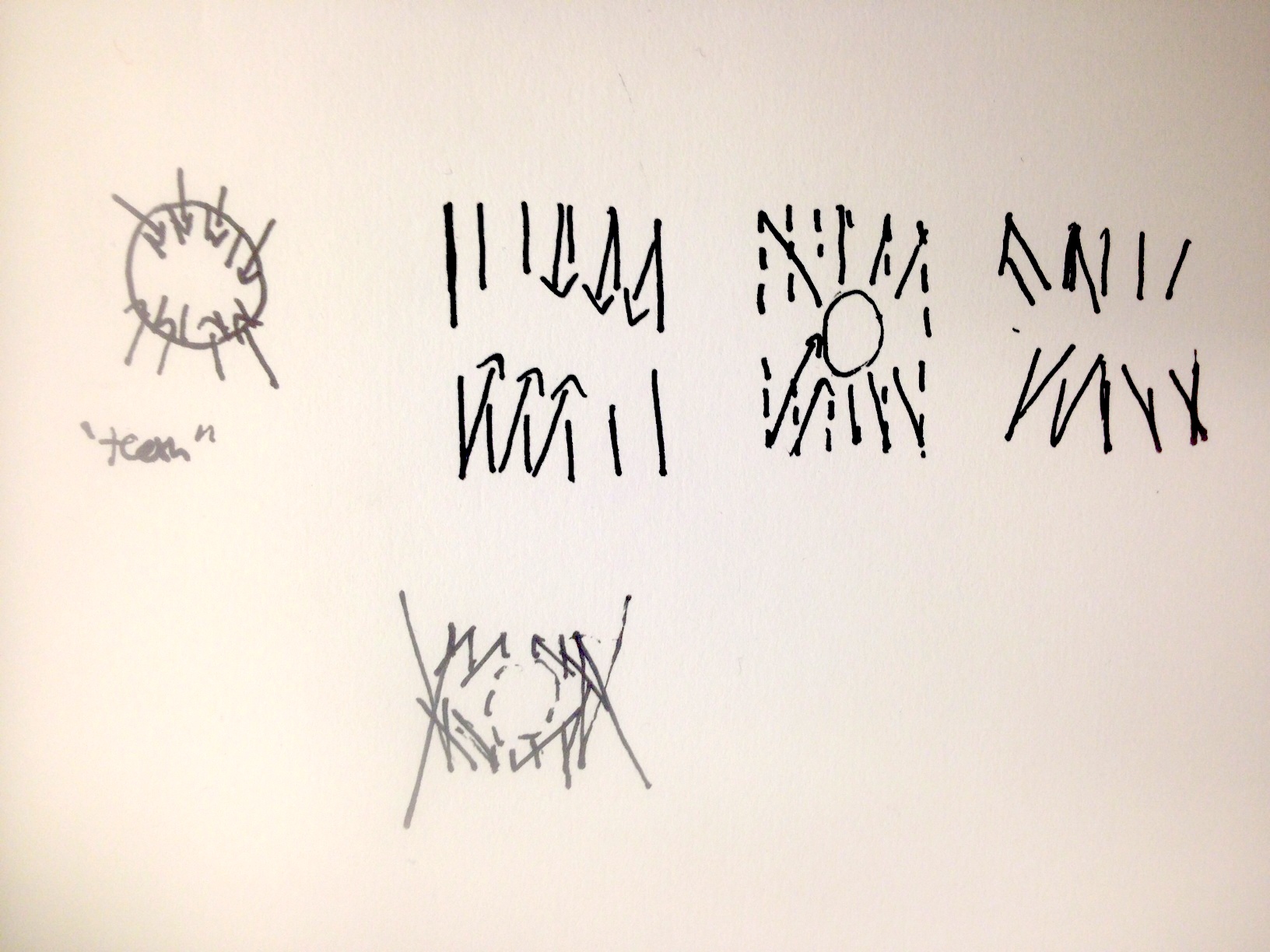
/**
* Register your submission and choose a character
* For more information check out the documentation
* http://anitype.com/documentation
*/
Anitype.register('O', {
// Enter your name
author: 'LValley',
// Enter a personal website, must have http
website: 'http://digital-love.squarespace.com/',
// Make your animation here
construct: function(two, points) {
// Reference to instance
var anitype = this;
//var x = 0;
var line_1 = two.makeLine(-700,329,-215,-329);
var line_2 = two.makeLine(-600,329, -215, -329);
var line_3 = two.makeLine(-500,329, -215, -329);
var line_4 = two.makeLine(-400,329,-215,-329);
var line_5 = two.makeLine(-300,329, -215, -329);
var line_6 = two.makeLine(-200,329, -215, -329);
var line_7 = two.makeLine(-100,329,-215,-329);
var line_8 = two.makeLine(0,329, -215, -329);
var line_9 = two.makeLine(100,329, -215, -329);
var line_10 = two.makeLine(200,329,-215,-329);
var line_11 = two.makeLine(300,329, -215, -329);
var line_12 = two.makeLine(400,329, -215, -329);
var line_13 = two.makeLine(500,329, -215, -329);
var line_14 = two.makeLine(600,329,-215,-329);
var line_15 = two.makeLine(700,329, -215, -329);
var line_16 = two.makeLine(800,329, -215, -329);
var line_17 = two.makeLine(900,329,-215,-329);
var line_18 = two.makeLine(1000,329, -215, -329);
var line_19 = two.makeLine(1100,329, -215, -329);
var line_20 = two.makeLine(-700, 0,-215,-329);
var line_21 = two.makeLine(-600, 0, -215, -329);
var line_22 = two.makeLine(-500, 0, -215, -329);
var line_23 = two.makeLine(-400,0,-215,-329);
var line_24 = two.makeLine(-300,0, -215, -329);
var line_25 = two.makeLine(-200,0, -215, -329);
var line_33 = two.makeLine(600,0,-215,-329);
var line_34 = two.makeLine(700,0, -215, -329);
var line_35 = two.makeLine(800,0, -215, -329);
var line_36 = two.makeLine(900,0,-215,-329);
var line_37 = two.makeLine(1000,0, -215, -329);
var line_38 = two.makeLine(1100,0, -215, -329);
var line_39 = two.makeLine(-700, -329,-215,-329);
var line_57 = two.makeLine(1100,-329, -215, -329);
var line_58 = two.makeLine(-700, -658,-215,-329);
var line_76 = two.makeLine(1100,-658, -215, -329);
var line_77 = two.makeLine(-700, -987,-215,-329);
var line_78 = two.makeLine(-600, -987, -215, -329);
var line_79 = two.makeLine(-500, -987, -215, -329);
var line_80 = two.makeLine(-400,-987,-215,-329);
var line_92 = two.makeLine(800,-987, -215, -329);
var line_93 = two.makeLine(900,-987,-215,-329);
var line_94 = two.makeLine(1000,-987, -215, -329);
var line_95 = two.makeLine(1100,-987, -215, -329);
//row_6
var line_96 = two.makeLine(-700, -1316,-215,-329);
var line_97 = two.makeLine(-600, -1316, -215, -329);
var line_98 = two.makeLine(-500, -1316, -215, -329);
var line_99 = two.makeLine(-400, -1316,-215,-329);
var line_100 = two.makeLine(-300,-1316, -215, -329);
var line_101 = two.makeLine(-200,-1316, -215, -329);
var line_102 = two.makeLine(-100,-1316,-215,-329);
var line_103 = two.makeLine(0,-1316, -215, -329);
var line_104 = two.makeLine(100,-1316, -215, -329);
var line_105 = two.makeLine(200,-1316,-215,-329);
var line_106 = two.makeLine(300,-1316, -215, -329);
var line_107 = two.makeLine(400,-1316, -215, -329);
var line_108 = two.makeLine(500,-1316, -215, -329);
var line_109 = two.makeLine(600,-1316,-215,-329);
var line_110 = two.makeLine(700,-1316, -215, -329);
var line_111 = two.makeLine(800,-1316, -215, -329);
var line_112 = two.makeLine(900,-1316,-215,-329);
var line_113 = two.makeLine(1000,-1316, -215, -329);
var line_114 = two.makeLine(1100,-1316, -215, -329);
line_1.vertices[0].set(0, 450);
line_1.vertices[1].set(0,350);
//line_2
line_2.vertices[0].set(0,450);
line_2.vertices[1].set(0,350);
//line_3
line_3.vertices[0].set(0,450);
line_3.vertices[1].set(0,350);
line_4.vertices[0].set(0,450);
line_4.vertices[1].set(0,350);
//line_2
line_5.vertices[0].set(0,450);
line_5.vertices[1].set(0,350);
//line_3
line_6.vertices[0].set(0,450);
line_6.vertices[1].set(0,350);
line_7.vertices[0].set(0, 450);
line_7.vertices[1].set(0,350);
//line_2
line_8.vertices[0].set(0,450);
line_8.vertices[1].set(0,350);
//line_3
line_9.vertices[0].set(0,450);
line_9.vertices[1].set(0,350);
line_10.vertices[0].set(0,450);
line_10.vertices[1].set(0,350);
//line_2
line_11.vertices[0].set(0,450);
line_11.vertices[1].set(0,350);
//line_3
line_12.vertices[0].set(0,450);
line_12.vertices[1].set(0,350);
//line_3
line_13.vertices[0].set(0,450);
line_13.vertices[1].set(0,350);
line_14.vertices[0].set(0,450);
line_14.vertices[1].set(0,350);
//line_2
line_15.vertices[0].set(0,450);
line_15.vertices[1].set(0,350);
//line_3
line_16.vertices[0].set(0,450);
line_16.vertices[1].set(0,350);
line_17.vertices[0].set(0, 450);
line_17.vertices[1].set(0,350);
//line_2
line_18.vertices[0].set(0,450);
line_18.vertices[1].set(0,350);
//line_3
line_19.vertices[0].set(0,450);
line_19.vertices[1].set(0,350);
//line_3
line_22.vertices[0].set(0,450);
line_22.vertices[1].set(0,350);
//line_3
line_23.vertices[0].set(0,450);
line_23.vertices[1].set(0,350);
line_24.vertices[0].set(0,450);
line_24.vertices[1].set(0,350);
//line_2
line_25.vertices[0].set(0,450);
line_25.vertices[1].set(0,350);
// //line_3
line_20.vertices[0].set(0,450);
line_20.vertices[1].set(0,350);
line_21.vertices[0].set(0, 450);
line_21.vertices[1].set(0,350);
//line_3
line_33.vertices[0].set(0,450);
line_33.vertices[1].set(0,350);
line_34.vertices[0].set(0,450);
line_34.vertices[1].set(0,350);
//line_2
line_35.vertices[0].set(0,450);
line_35.vertices[1].set(0,350);
line_39.vertices[0].set(0,450);
line_39.vertices[1].set(0,350);
// //line_2
line_36.vertices[0].set(0,450);
line_36.vertices[1].set(0,350);
// //line_3
line_37.vertices[0].set(0,450);
line_37.vertices[1].set(0,350);
// //line_3
line_38.vertices[0].set(0,450);
line_38.vertices[1].set(0,350);
line_57.vertices[0].set(0, 450);
line_57.vertices[1].set(0,350);
// //line_2
line_58.vertices[0].set(0,450);
line_58.vertices[1].set(0,350);
// //line_3
line_76.vertices[0].set(0,450);
line_76.vertices[1].set(0,350);
line_77.vertices[0].set(0, 450);
line_77.vertices[1].set(0,350);
//line_2
line_78.vertices[0].set(0,450);
line_78.vertices[1].set(0,350);
line_79.vertices[0].set(0,450);
line_79.vertices[1].set(0,350);
line_80.vertices[0].set(0,450);
line_80.vertices[1].set(0,350);
line_92.vertices[0].set(0,450);
line_92.vertices[1].set(0,350);
// //line_3
line_93.vertices[0].set(0,450);
line_93.vertices[1].set(0,350);
line_94.vertices[0].set(0,450);
line_94.vertices[1].set(0,350);
//line_2
line_95.vertices[0].set(0,450);
line_95.vertices[1].set(0,350);
//line_3
line_96.vertices[0].set(0,450);
line_96.vertices[1].set(0,350);
line_97.vertices[0].set(0, 450);
line_97.vertices[1].set(0,350);
//line_2
line_98.vertices[0].set(0,450);
line_98.vertices[1].set(0,350);
//line_3
line_99.vertices[0].set(0,450);
line_99.vertices[1].set(0,350);
line_100.vertices[0].set(0,450);
line_100.vertices[1].set(0,350);
//line_2
line_101.vertices[0].set(0,450);
line_101.vertices[1].set(0,350);
//line_3
line_102.vertices[0].set(0,450);
line_102.vertices[1].set(0,350);
//line_3
line_103.vertices[0].set(0,450);
line_103.vertices[1].set(0,350);
line_104.vertices[0].set(0,450);
line_104.vertices[1].set(0,350);
//line_2
line_105.vertices[0].set(0,450);
line_105.vertices[1].set(0,350);
//line_3
line_106.vertices[0].set(0,450);
line_106.vertices[1].set(0,350);
line_107.vertices[0].set(0, 450);
line_107.vertices[1].set(0,350);
//line_2
line_108.vertices[0].set(0,450);
line_108.vertices[1].set(0,350);
//line_3
line_109.vertices[0].set(0,450);
line_109.vertices[1].set(0,350);
line_110.vertices[0].set(0,450);
line_110.vertices[1].set(0,350);
//line_2
line_111.vertices[0].set(0,450);
line_111.vertices[1].set(0,350);
//line_3
line_112.vertices[0].set(0,450);
line_112.vertices[1].set(0,350);
//line_3
line_113.vertices[0].set(0,450);
line_113.vertices[1].set(0,350);
line_114.vertices[0].set(0,450);
line_114.vertices[1].set(0,350);
//Create the animation via a tween
function moveVert(vert, x1, x2, y1, y2,start,duration){
anitype.addTween(line_5, {
to: {x :0, y : -1000 },
easing: Anitype.Easing.Linear.Out,
update: function() {
anitype.addTween(vert, {
to: {x: x2, y:y2},
easing: Anitype.Easing.Elastic.Out,
duration: duration,
start: start
});
},
duration: duration, // Value from 0 - 1
start: start // Value from 0 - 1
});
}
//Create the animation via a tween
function moveVert1(vert, x1, x2, y1, y2,start,duration){
anitype.addTween(line_3, {
to: { x: 0, y: 1000 },
easing: Anitype.Easing.Linear.Out,
update: function() {
anitype.addTween(vert, {
to: {x: x2, y:y2},
easing: Anitype.Easing.Elastic.Out,
duration: duration,
start: start
});
},
duration: duration, // Value from 0 - 1
start: start // Value from 0 - 1
});
}
//moveVert(line_1.vertices[1],0,215,-329,-329,0,.5);
//moveVert(line_10.vertices[1],0,-215,-329,-100,.25,.75);
moveVert(line_3.vertices[1],0,-215,-329,-800,.4,.75);
moveVert(line_5.vertices[1],0,-215,-329,-250,.4,.45);
moveVert(line_7.vertices[1],0,-215,-329,70,.2,.75);
moveVert(line_9.vertices[1],0,-215,-329,250,.2,.75);
moveVert(line_11.vertices[1],0,215,-329,250,.2,.75);
moveVert(line_13.vertices[1],0,215,-329,0,.2,.75);
moveVert(line_15.vertices[1],0,215,-329,-250,.4,.75);
moveVert(line_17.vertices[1],0,215,-329,-800,.4,.75);
//moveVert(line_19.vertices[1],0,-215,-329,-800,.25,.5);
moveVert(line_22.vertices[1],0,-215,-329,-250,.5,.75);
moveVert(line_24.vertices[1],0,-215,-329,70,.5,.75);
moveVert(line_25.vertices[1],0,-215,-329,250,.5,.75);
moveVert(line_33.vertices[1],0,215,-329,250,.5,.75);
moveVert(line_34.vertices[1],0,215,-329,0,.5,.75);
moveVert(line_35.vertices[1],0,215,-329,-250,.5,.75);
//top
//moveVert(line_1.vertices[1],0,215,-329,-329,0,.5);
//moveVert(line_10.vertices[1],0,-215,-329,-100,.25,.75);
moveVert(line_104.vertices[0],0,-215,-329,550,.2,.75);
moveVert(line_106.vertices[0],0,215,-329,550,.2,.75);
moveVert(line_102.vertices[0],0,-215,-329,700,.2,.75);
moveVert(line_108.vertices[0],0,215,-329,700,.2,.75);
moveVert(line_100.vertices[0],0,-215,-329,900,.5,.75);
moveVert(line_110.vertices[0],0,215,-329,900,.5,.75);
moveVert(line_98.vertices[0],0,-215,-329,1100,.5,.75);
moveVert(line_112.vertices[0],0,215,-329,1100,.25,.75);
moveVert(line_78.vertices[0],0,-215,-329, 1300,.4,.75);
moveVert(line_94.vertices[0],0,215,-329,1300,.4,.75);
// Return your polygon wrapped in a group.
return two.makeGroup(line_1,line_2, line_3, line_4, line_5, line_6, line_7, line_8, line_9, line_10, line_11, line_12, line_13, line_14, line_15, line_16, line_17, line_18, line_19, line_20, line_21, line_22, line_23,line_24, line_25, line_33, line_34, line_35, line_36, line_37, line_38, line_39, line_57, line_58, line_76, line_77, line_78, line_79, line_80,line_92, line_93, line_94, line_95, line_96, line_97, line_98, line_99, line_100, line_101, line_102, line_103,line_104, line_105, line_106, line_107, line_108, line_109, line_110, line_111, line_112, line_113, line_114);
}
});