“My program parametrically generates knit patterns for the Disney Research knitting machine”
I decided to make use of the industrial knitting machine at Disney Research Pittsburgh. The native interface to the machine lacks a lot of abstraction — it takes in a series of machine instructions — which needle/bed to loop on, direction of knit, transfers from one needle to another, etc. — and executes them in order. Researchers have built a small layer on top that translates these instructions to JavaScript, but the layer of abstraction remains pretty thin. Essentially, in order to knit something with the knitting machine, you need to translate your vision for the garment into a list of instructions to the knitting machine, which is both tricky to get right and very hard to generalize.
This gulf between vision and execution is what inspired me to try and create a parametric knit object. Working so closely with machine primitives would make it challenging, because it would require building up some generalizable (and easily parameterized) abstractions, as well as making sure the patterns were physically feasible, and the instructions, when executed, would result in a well-formed, knittable object. Unfortunately, this also means the machine lacks realistic renderings, and so output cannot be visualized until it is fed into the machine. Currently, I’m not posting the output files, because I’m not sure whether Disney considers them proprietary. I’ll find out.
I’d knit some simple patterns with the machine before, and spent a lot of time trying to figure out what forms could be generalized without everything breaking. Originally, I was interested in trying to make hats, but the process of making a closed tube on the machine ended up being much too complicated to easily generalize. After consulting with other researchers, I decided that a good first step would be to create generative patterns on flat, knit rectangles.

Cables
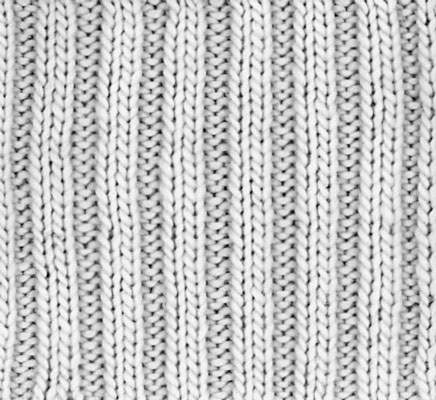
Ribs
A lot of the cooler knit patterns seemed to be formed out of “ribs” (raised lines of purl stitches) and “cables” (crossed purls that form a braid). Alone, they were pretty simple to knit, but when used effectively, they could be combined to create some pretty elaborate looking patterns:
My first step, then, was to create generalized abstractions for ribs and cables. After a lot of experimentation, I came up with two parametric forms (both allow the width and height of the knit object to be specified):

Sketch of pattern 1
1. The first pattern generates n vertical ribs spanning the height of the pattern. The ribs curve in random directions, and have an “affinity” for one another: when two ribs come within a certain distance horizontally, they merge and combine as a single cable.
While the pattern itself was simple, figuring out exactly when two ribs would collide is actually quite tricky, and curved ribs “colliding” with one another easily breaks a pattern when handled wrong. Fixing this required a lot of ugly code to check updates, and treat sufficiently close ribs as a single, larger rib.

Sketch of pattern 2

Example of the knit output from pattern 2
2. The second pattern has straight, vertical ribs evenly spaced across the pattern, and generative places cross-cables in random locations to “bridge” them. While simpler and mostly lacking in the collision problem, I found this pattern to be more aesthetically pleasing than the other one. I suppose there’s something to be said about simplicity.
Disney researchers are still in the process of building up abstractions for the knitting machine that will allow one to specify a garment as a program. I’m glad I was able to contribute to the space by create some parameterized patterns that try to make generalizable patterns out of primitive instructions. I think there’s still a lot of work to be done in this area, and I’d be interested in learning more about what common patterns can be generalized without breaking down in execution.